In C language, arrays are referred to as structured data types. An array is a collection of data that holds fixed number of values of same data type.
Arrays store a fixed-size sequential collection of elements of the same type. In other words, if we want to group similar type of value in conjunctive memory location then we use Array.
Points :
Arrays store a fixed-size sequential collection of elements of the same type. In other words, if we want to group similar type of value in conjunctive memory location then we use Array.
Points :
- Array might be belonging to any of the data types
- Array size must be a constant value.
- Always, adjacent memory locations are used to store array elements in memory.
- Array should be initialized with zero or null while declaring, if we don’t assign any values to array.
The size and type of arrays cannot be changed after its declaration.
Arrays are of two types:
- One-dimensional arrays
- Multidimensional arrays
One-dimensional Arrays :
Group of values in adjacent memory location in x or y direction is 1-D array.
Declaration of One-dimensional array :
data_type array_name[array_size];
For example :
float val[10];
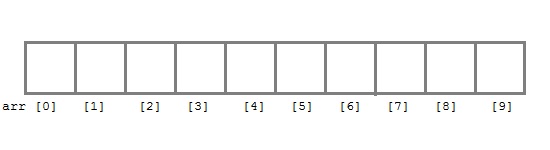
Here, we declared an array, val of floating-point type and size 10. Meaning, it can hold 10 floating-point values.
Key notes:
- Arrays have 0 as the first index. In this example, val[0]
- If the size of an array is n, then last element will be (n-1). In this example, val[9]
- Suppose the starting address of val[0] is 2124d. Then, the next address, val[1], will be 2128d, address of val[2] will be 2132d and so on. Because size of float data type is 4 bytes.
Initialization of 1-D Array :
After an array is declared, it’s elements must be initialized. In C programming an array can be initialized at either of the following stages:
- At compile time
- At run time
Compile time initialization :
Compile time initialization of array elements is same as normal variable initialization. The general form of initialization of array is :
type array-name[size] = { list of values };
The values in the list are separated by commas. For ex, the statement
int number[3] = { 0,5,4 };
will declare the variable’ number’ as an array of size 3 and will assign the values to each element. If the number of values in the list is less than the number of elements, then only that many elements will be initialized. The remaining elements will be set to zero automatically.
Remember, if we have more initializers than the declared size, the compiler will produce an error.
Example :
#include <stdio.h>
int main () {
int n[ 10 ] ; /* n is an array of 10 integers */
int i, j ;
/* initialize elements of array n to 0 */
for ( i = 0; i < 10; i++ ) {
n[ i ] = i + 100; /* set element at location i to i + 100 */
}
/* output each array element's value */
for (j = 0; j < 10; j++ ) {
printf("Element[%d] = %d\n", j, n[j] );
}
return 0;
}
Output :
Element[0] = 100
Element[1] = 101
Element[2] = 102
Element[3] = 103
Element[4] = 104
Element[5] = 105
Element[6] = 106
Element[7] = 107
Element[8] = 108
Element[9] = 109
Run time initialization :
An array can also be initialized at runtime using scanf() function. This approach is usually used for initializing large array, or to initialize array with user specified values.
Example :
#include<stdio.h>
int main()
{
int array[5],i;
printf("Enter 5 numbers to store them in array : \n");
for(i=0;i<5;i++)
{
scanf("%d",&array[i]);
}
printf("Element in the array are : \n \n");
for(i=0;i<5;i++)
{
printf("Element stored at a[%d] : %d \n",i,array[i]);
}
return 0;
}
Output :
Enter 5 elements in the array : 23 45 32 25 45
Elements in the array are :
Element stored at a[0] : 23
Element stored at a[0] : 45
Element stored at a[0] : 32
Element stored at a[0] : 25
Element stored at a[0] : 45
Multi dimension Array :
In C, we can define multidimensional arrays in simple words as array of arrays. Data in multidimensional arrays are stored in tabular form.
General form of declaring N-dimensional arrays:
data_type array_name[size1][size2]....[sizeN];
where,
data_type: Type of data to be stored in the array.
Here data_type is valid C/C++ data type
array_name: Name of the array
size1, size2,... ,sizeN: Sizes of the dimensions
Two dimensional Array :
The simplest form of multidimensional array is the two-dimensional array. A two-dimensional array is, in essence, a list of one-dimensional arrays.
Declaration :
type array-name[row-size][column-size]
int a[3][4];
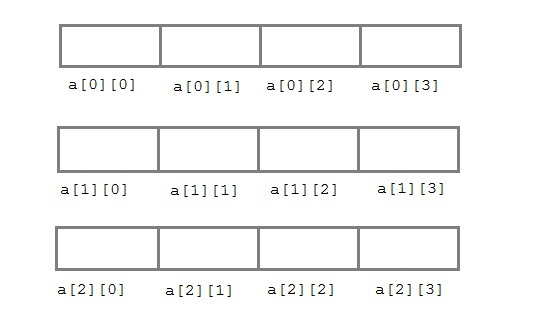
Initialization :
int a[3][4] = {
{0, 1, 2, 3} , /* initializers for row indexed by 0 */
{4, 5, 6, 7} , /* initializers for row indexed by 1 */
{8, 9, 10, 11} /* initializers for row indexed by 2 */
};
int a[3][4] = {0,1,2,3,4,5,6,7,8,9,10,11};
Example :
#include <stdio.h>
int main () {
/* an array with 5 rows and 2 columns*/
int a[5][2] = { {0,0}, {1,2}, {2,4}, {3,6},{4,8}};
int i, j;
/* output each array element's value */
for ( i = 0; i < 5; i++ ) {
for ( j = 0; j < 2; j++ ) {
printf("a[%d][%d] = %d\n", i,j, a[i][j] );
}
}
return 0;
}
Output :
a[0][0]: 0
a[0][1]: 0
a[1][0]: 1
a[1][1]: 2
a[2][0]: 2
a[2][1]: 4
a[3][0]: 3
a[3][1]: 6
a[4][0]: 4
a[4][1]: 8
Three dimensional Array :
Three-Dimensional array is same as that of Two-dimensional arrays. The difference is as the number of dimension increases so the number of nested braces will also increase.
Initialization of a three dimensional array :
You can initialize a three dimensional array in a similar way like a two dimensional array. Here's an example,
int test[2][3][4] = {
{ {3, 4, 2, 3}, {0, -3, 9, 11}, {23, 12, 23, 2} },
{ {13, 4, 56, 3}, {5, 9, 3, 5}, {3, 1, 4, 9} }
};
Example :
#include<iostream>
using namespace std;
int main()
{
// initializing the 3-dimensional array
int x[2][3][2] =
{
{ {0,1}, {2,3}, {4,5} },
{ {6,7}, {8,9}, {10,11} }
};
// output each element's value
for (int i = 0; i < 2; ++i)
{
for (int j = 0; j < 3; ++j)
{
for (int k = 0; k < 2; ++k)
{
cout << "Element at x[" << i << "][" << j
<< "][" << k << "] = " << x[i][j][k]
<< endl;
}
}
}
return 0;
}
Output:
Element at x[0][0][0] = 0
Element at x[0][0][1] = 1
Element at x[0][1][0] = 2
Element at x[0][1][1] = 3
Element at x[0][2][0] = 4
Element at x[0][2][1] = 5
Element at x[1][0][0] = 6
Element at x[1][0][1] = 7
Element at x[1][1][0] = 8
Element at x[1][1][1] = 9
Element at x[1][2][0] = 10
Element at x[1][2][1] = 11