Case control statements are used to execute only specific block of statements in a series of block.
Flowchart of break statement :
Types of Case Control Statements :
- switch
- break
- continue
switch :
Switch case statements are a substitute for long if statements that compare a variable to several "integral" values The
if..else..if
ladder allows you to execute a block code among many alternatives. If you are checking on the value of a single variable in if...else...if
, it is better to use switch statement. The switch statement is often faster than nested if...else
(not always).
The value of the variable given into switch is compared to the value following each of the cases, and when one value matches the value of the variable, the computer continues executing the program from that point.
Syntax :
switch(expression)
{
case value-1:
block-1;
break;
case value-2:
block-2;
break;
case value-3:
block-3;
break;
case value-4:
block-4;
break;
default:
default-block;
break;
}
It evaluates the value of expression or variable (based on whatever is given inside switch braces), then based on the outcome it executes the corresponding case.
switch Statement Flowchart :
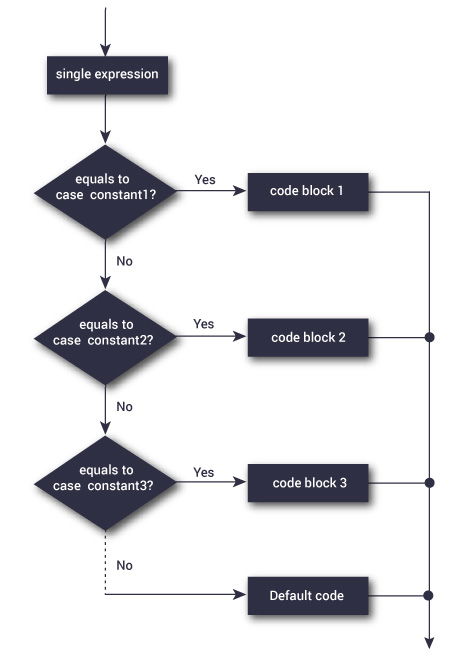
Example :
#include <stdio.h>
int main () {
/* local variable definition */
char grade = 'B';
switch(grade) {
case 'A' :
printf("Excellent!\n" );
break;
case 'B' :
case 'C' :
printf("Well done\n" );
break;
case 'D' :
printf("You passed\n" );
break;
case 'F' :
printf("Better try again\n" );
break;
default :
printf("Invalid grade\n" );
}
printf("Your grade is %c\n", grade );
return 0;
}
Output :
Well done
Your grade is B
break Statement :
Break statement is used to terminate the while loops, switch case loops and for loops from the subsequent execution. The break statement is used with decision making statement such as if..else.
Syntax :
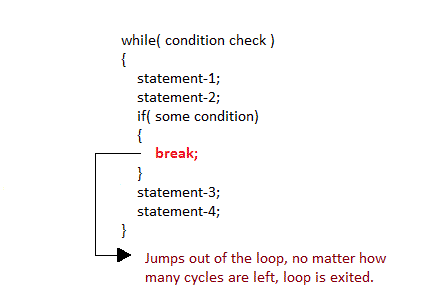
Flowchart of break statement :
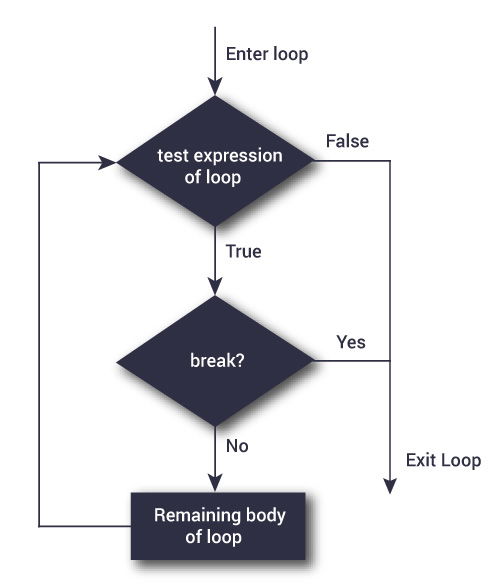
Example :
#include <stdio.h>
int main()
{
int i;
for(i=0;i<10;i++)
{
if(i==5)
{
printf("\n Coming out of for loop when i = 5");
break;
}
printf("%d ",i);
}
}
Output :
0 1 2 3 4
Coming out of for loop when i = 5
continue Statement :
Continue statement is used to continue the next iteration of for loop, while loop and do-while loops. So, the remaining statements are skipped within the loop for that particular iteration.
Continue statement is mostly used inside loops. Whenever it is encountered inside a loop, control directly jumps to the beginning of the loop for next iteration, skipping the execution of statements inside loop’s body for the current iteration.
Syntax :
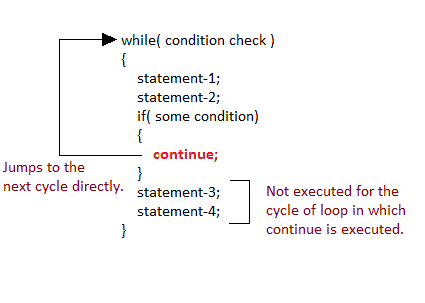
Flowchart of continue Statement :
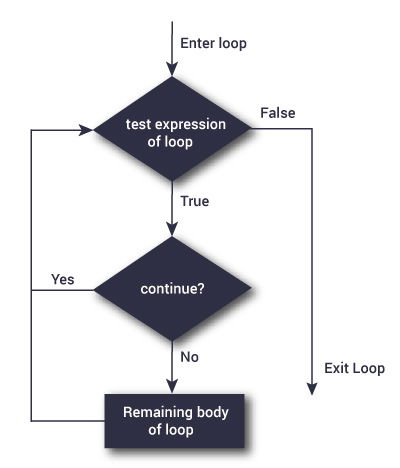
Example :
#include <stdio.h>
int main()
{
int i;
for(i=0;i<10;i++)
{
if(i==5 || i==6)
{
printf("\nSkipping %d from display using continue statement \n",i);
"continue statement \n",i);
continue;
}
printf("%d ",i);
}
}
Output:
0 1 2 3 4
Skipping 5 from display using continue statement
Skipping 6 from display using continue statement
7 8 9