Loops are used in programming to repeat a specific block of code. You may encounter situations, when a block of code needs to be executed several number of times. In general, statements are executed sequentially: The first statement in a function is executed first, followed by the second, and so on.
In any programming language, loops are used to execute a set of statements repeatedly until a particular condition is satisfied.
Flowchart :

Types of Loop :
There are 3 types of loop control statements in C language. They are,
In any programming language, loops are used to execute a set of statements repeatedly until a particular condition is satisfied.
Flowchart :

Types of Loop :
There are 3 types of loop control statements in C language. They are,
- for
- while
- do-while
for loop :
for loop is used to execute a set of statements repeatedly until a particular condition is satisfied. we can say it an open ended loop.
Syntax :
for ( init; condition; increment ) {
statement(s);
}
for loop Flowchart :
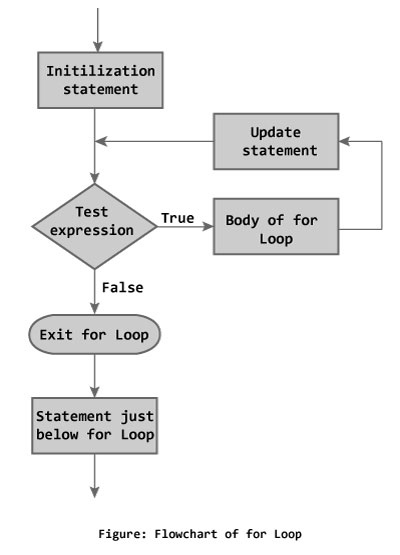
In for loop we have exactly two semicolons, one after initialization and second after condition. In this loop we can have more than one initialization or increment/decrement, separated using comma operator. for loop can have only one condition.
The initialization statement is executed only once.Then, the test expression is evaluated. If the test expression is false (0), for loop is terminated. But if the test expression is true (nonzero), codes inside the body of for loop is executed and the update expression is updated.
Example :
#include <stdio.h>
int main()
{
int i;
for(i=0;i<10;i++) {
printf("%d ",i);
}
}
Output :
0 1 2 3 4 5 6 7 8 9 9
Nested for loop :
We can also have nested for loops, i.e one for loop inside another for loop.
Syntax :
for(initialization; condition; increment/decrement)
{
for(initialization; condition; increment/decrement)
{
statement ;
}
}
while loop :
while loop can be addressed as an entry control loop, in which statements are executed only if condition is true. It is completed in 3 steps.
- Variable initialization.( e.g int a=0; )
- condition( e.g while( a<=10) )
- Variable increment or decrement ( a++ or a-- or a=a+2 )
Syntax :
variable initialization ;
while (condition)
{
statements ;
variable increment/decrement ;
}
Flowchart of while loop :
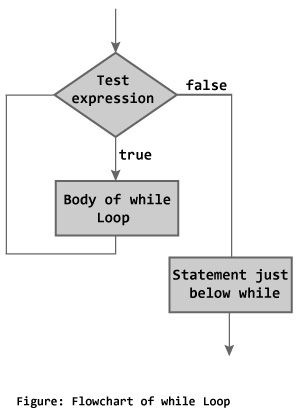
The while loop evaluates the test expression. If the test expression is true (nonzero), codes inside the body of while loop are executed. The test expression is evaluated again. The process goes on until the test expression is false. When the test expression is false, the while loop is terminated.
Example :
#include <stdio.h>
int main()
{
int i=3;
while(i<10)
{
printf("%d\n",i);
i++;
}
}
OUTPUT:
3 4 5 6 7 8 9
do while loop :
Unlike while, do while is an exit controlled loop. Here the set of statements inside braces are executed first. The condition inside while is checked only after finishing the first time execution of statements inside braces. If the condition is TRUE, then statements are executed again. This process continues as long as condition is TRUE. Program control exits the loop once the condition turns FALSE.
Syntax :
do
{
....
.....
}
while(condition)
Flowchart of do while loop :
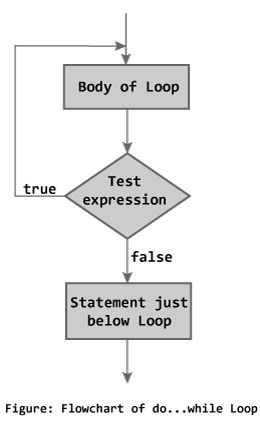
Example :
#include <stdio.h>
int main()
{
int i=1;
do
{
printf("Value of i is %d\n",i);
i++;
}
while(i<=4 && i>=2);
}
Output:
Value of i is 1
Value of i is 2
Value of i is 3
Value of i is 4
Difference between while and do while loop :
while | do while |
Loop is executed only when condition is true.
| Loop is executed for first time irrespective of the condition. After executing while loop for first time, then condition is checked. |